Software System
Audio Analysis
Minim
To do audio analysis, we use the Processing language and IDE, and
the Minim library.
Minim allows us to open audio files using minim.loadFile()
.
It also provides the BeatDetect
and BeatListener
classes that provide real-time audio analysis with its API through
these methods:
beatListener.isKick()
beatListener.isSnare()
beatListener.isHat()
beatListener.isOnset(int beat)
We arbitrarily choose to check if every sixth beat is onset, so we would
call beatListener.isOnset(5)
.
Communication
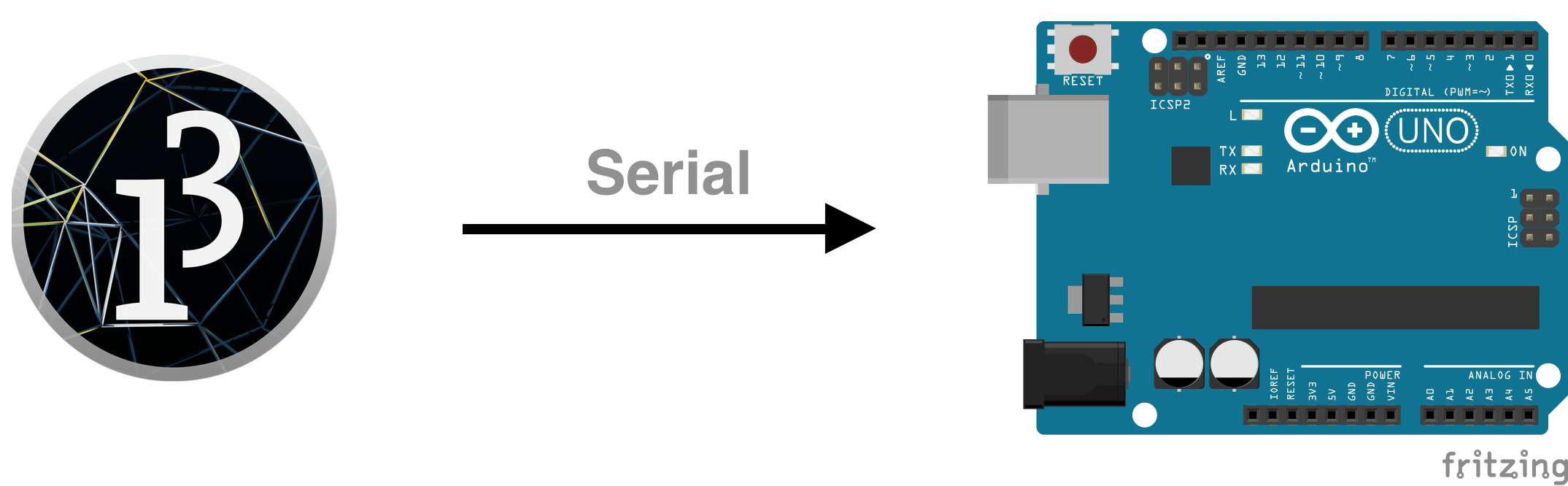
In order to have Processing communicate with the Arduino, we use
Processing's built-in Serial
class to establish the
connection. All of this goes in the setup()
function, in
which we extract the portname and connect to it with:
String portName = Serial.list()[1];
myPort = new Serial(this, portName, 9600):
Encoding
Each of the four beats (kick, snare, hat and beat onset) corresponds to a certain LED pattern.
In order to tell the Arduino which pattern to light up, we encode each type of
beat detected as a bit in a 4-bit message. For example, 1100
means
there was a kick, a snare, no hat and no sixth beat onset.
Once connected to Serial, each time through draw()
the program calls
the aforementioned BeatListener
methods and if any returns true,
it encodes the corresponding bit as 1
. This is equivalent to adding
the decimal equivalent of that beat type to a sum
variable. The logic
is as follows:
int msg = 0;
if (beat.isKick()) msg += 1;
if (beat.isSnare()) msg += 2;
if (beat.isHat()) msg += 4;
if (beat.isOnset(5)) msg += 8;
myPort.write(msg.toString() + "x");
The suffixed "x"
is to signal to the Arduino the end of the message. The Arduino
reads this message with Serial.readStringUntil('x').toInt()
.