Software Subsystem
We had two primary pieces to our software subsystem - low-level firmware that ran on the Arduino and high-level software that ran on the Intel Euclid. The high-level software used ROS to connect the various parts of the project together and dealt with things like person tracking, switching between robot states, and providing an interface for the user to work with while the low-level firmware dealt with sending appropriate PWM values to the motors in order to execute the high-level commands. This page describes in detail the software architecture at work to make our project run. All of our code as well as dependencies and setup instructions can be found on our GitHub.
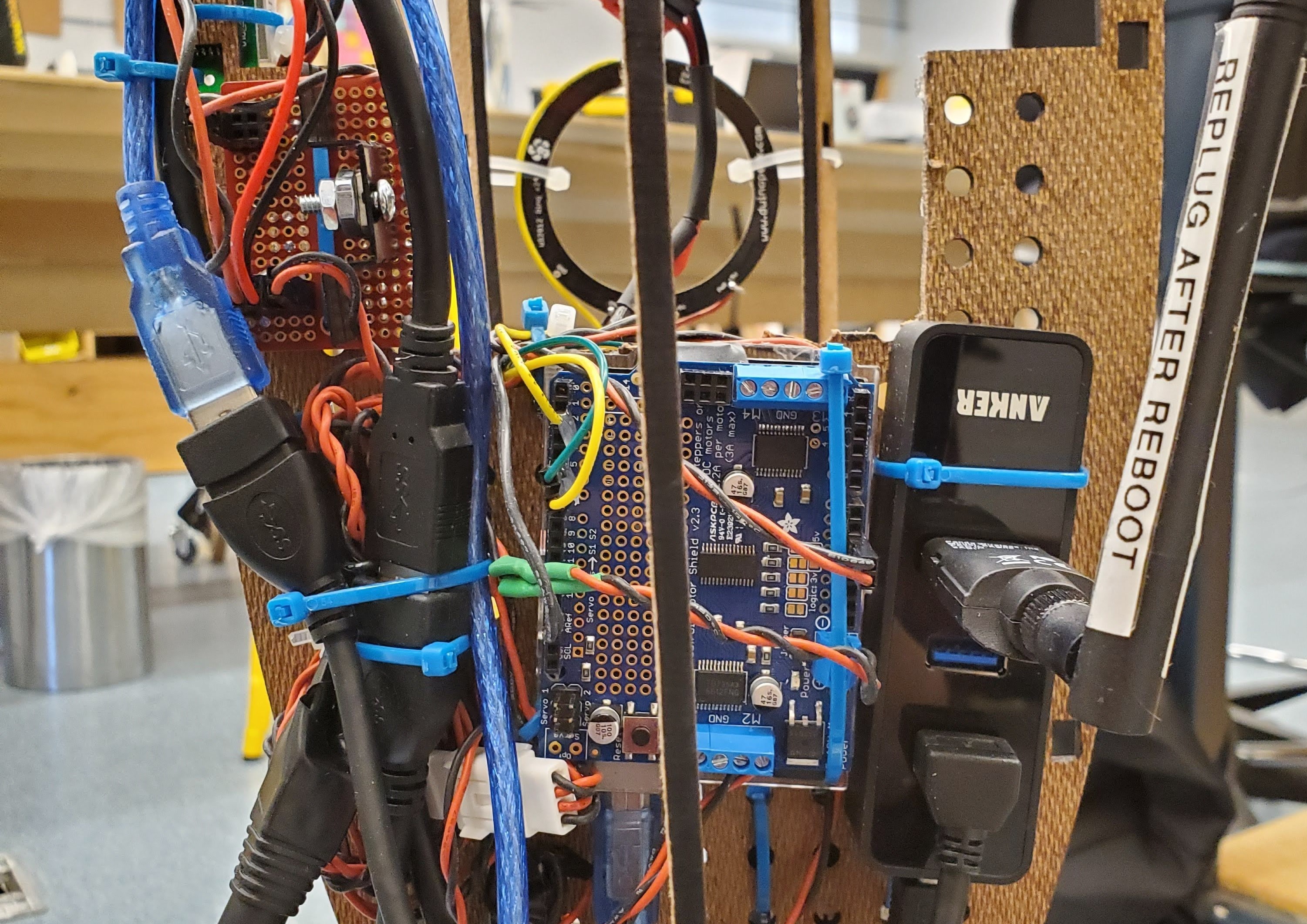
Low-level Firmware
The firmware we wrote can be viewed in the src folder of our repo.
Here is a list of files and their function:
msgs.h - contains a data structure to save the robot commands in both a numerical and a string format
driveBase.h/.cpp - contains a class for interfacing with the robot. Basically translates an incoming message in the format listed in msgs.h to valid PWM values for the robot. A proportional controller for each wheel can also be found in this file.
turret_base.h/.ino - the main Arduino code. Listens for serial input from the high-level code and parses the input to create a properly formatted robotCmd message (format is listed in msgs.h). Passes this message along to the DiffDriveRobot class to move the motors
High-level Software
This is how the software works for the POEtal Turret.
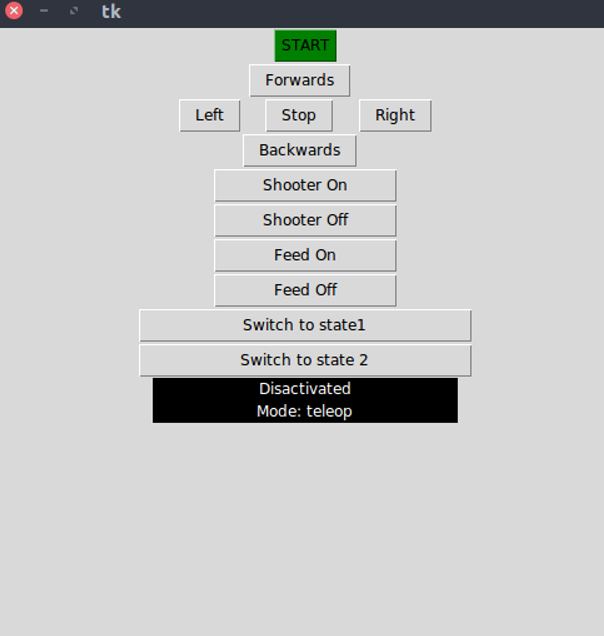
1. The user clicks a button on the GUI
2. On the backend, the GUI updates the ROS topic /state with what the current state of the robot should be
3. On the backend, there is a state controller continuously listening to /state for updates to the robot’s state
4. For simple states, the state controller calls the appropriate behavior function
5. The behavior function outputs a properly formatted command
6. The state controller publishes that command to the ROS topic /cmd_vel
7. On the backend, there is a script continuously listening to the topic /cmd_vel and reformatting those messages to arduino serial commands and sending them to the Arduino.
8. For more complex behaviors like the following behavior and the joystick teleoperation behavior, these commands each have scripts that continuously run in the background. Each of these scripts has its own ROS node and they each publish commands labeled with their appropriate state to /state_controller/cmd_vel.
9. For the more complex behaviors, instead of calling a behavior function when the state matches, it forwards the appropriate commands from /state_controller/cmd_vel to /cmd_vel